- Published on
Getting Started with Solana Development
- Authors
- Name
- The Alchemist
- @dnwemedia
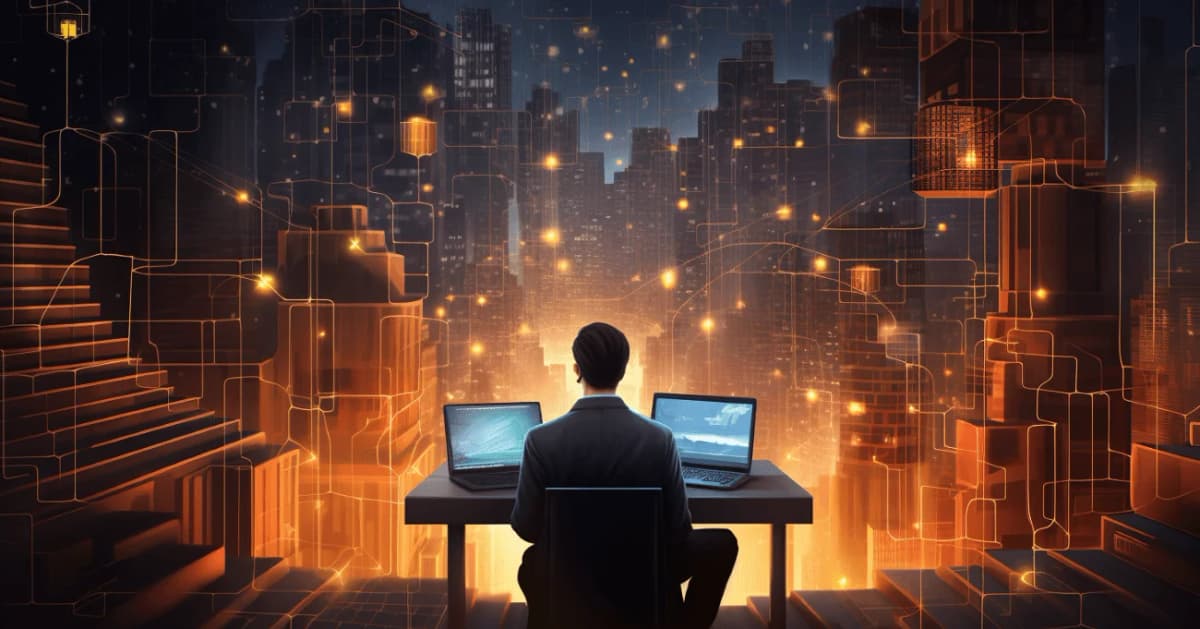
Learn how to get started with Solana development, from setting up your environment to building onchain programs and client-side dApps. This comprehensive guide covers everything you need to know to start building on the Solana blockchain.
Table of Contents
- Introduction to Solana Development
- High-Level Overview of Solana Development
- Onchain Program Development
- Client-Side Development
- Essential Tools for Solana Development
- Tools for Client-Side Development
- Tools for Onchain Program Development
- Setting Up Your Development Environment
- Installing Rust
- Setting Up Solana CLI
- Running a Local Validator
- Introduction to Solana Playground
- Building on Solana with Anchor
- Native Rust vs. Anchor Framework
- Testing Your Solana Programs
- Developer Environments on Solana
- Mainnet Beta
- Devnet
- Local Environment
- Building by Example: Resources and Guides
- Solana Cookbook
- Solana Program Examples
- Solana Guides
- Frequently Asked Questions
- What programming languages can I use for Solana development?
- Do I need to learn Rust to develop on Solana?
- What is the Solana CLI, and why do I need it?
- Can I test my Solana programs locally?
- What is the difference between native Rust and Anchor on Solana?
Introduction to Solana Development
Solana is a high-performance blockchain platform that has gained popularity among developers for its speed, scalability, and low transaction costs. Whether you're new to blockchain development or an experienced developer looking to explore Solana, this guide will provide you with a comprehensive overview of how to get started with Solana development.
In this article, we'll cover everything from the basic requirements and tools you'll need to the different types of development you can do on Solana. By the end of this guide, you'll be well-equipped to start building on the Solana blockchain.
High-Level Overview of Solana Development
Onchain Program Development
Onchain program development on Solana involves creating custom programs that are deployed directly to the blockchain. These programs, once deployed, can be interacted with by anyone who knows how to communicate with them. You can write these programs in Rust, C, or C++, with Rust being the most supported language for onchain development on Solana.
Onchain programs on Solana are highly composable, meaning you can build on top of existing programs without needing to create custom onchain logic. For example, if you want to work with tokens, you can utilize the pre-existing Token Program on Solana, which handles most of the onchain logic for you.
Client-Side Development
Client-side development on Solana involves creating decentralized applications (dApps) that interact with onchain programs. Unlike traditional development, where the backend is hosted on centralized servers, Solana's backend is a global, permissionless blockchain. This means that anyone can interact with your onchain program without the need for API keys or other forms of permission.
The Solana JSON RPC API acts as the "glue" between the client side and the onchain side. It allows your dApps to submit transactions and interact with onchain programs, similar to how a frontend interacts with a backend in traditional development.
Essential Tools for Solana Development
Tools for Client-Side Development
For client-side development on Solana, you can use any programming language you're comfortable with. Solana offers community-contributed SDKs to help developers interact with the Solana network in popular languages such as:
- Rust:
solana_sdk
- Typescript:
@solana/web3.js
- Python:
solders
- Java:
solanaj
- C++:
solcpp
- Go:
solana-go
- Kotlin:
solanaKT
- Dart:
solana
To quickly get started with a front-end for your application, you can generate a customizable Solana scaffold using the following command in your CLI:
npx create-solana-dapp <project-name>
This command creates a new project with all the necessary files and basic configuration to start building on Solana. The scaffold includes both an example frontend and an onchain program template (if selected).
Tools for Onchain Program Development
Onchain program development on Solana requires a few specific tools. First, you'll need to install Rust on your machine, which you can do with the following command:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
Next, you'll need to install the Solana CLI to compile and deploy your programs:
sh -c "$(curl -sSfL https://release.anza.xyz/stable/install)"
For testing your programs, it's recommended to run a local validator on your machine using the following command:
solana-test-validator
Running a local validator allows you to test your programs in a controlled environment before deploying them to a live network.
For developers who prefer not to develop their programs locally, Solana Playground offers an online IDE where you can write, test, and deploy programs on Solana.
Setting Up Your Development Environment
Installing Rust
Rust is the primary language for onchain program development on Solana. To install Rust, use the following command:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
This will install Rust on your machine, allowing you to begin writing and compiling onchain programs for Solana.
Setting Up Solana CLI
The Solana CLI is a command-line tool that allows you to compile, deploy, and interact with your onchain programs. To install the Solana CLI, run the following command:
sh -c "$(curl -sSfL https://release.anza.xyz/stable/install)"
Once installed, the Solana CLI provides a suite of commands to manage your onchain programs and interact with the Solana network.
Running a Local Validator
Before deploying your programs to the live network, it's essential to test them in a local environment. You can start a local validator on your machine by running:
solana-test-validator
This command sets up a local version of the Solana network, allowing you to test your programs in a safe environment.
Introduction to Solana Playground
Solana Playground is an online IDE that simplifies the development process by providing a web-based environment to write, test, and deploy Solana programs. It's a great tool for developers who prefer not to set up a local development environment.
Building on Solana with Anchor
Native Rust vs. Anchor Framework
When building on Solana, you have the choice of writing your programs in native Rust or using the Anchor framework. Anchor provides a higher-level API that simplifies the development process, similar to how React simplifies web development compared to raw JavaScript and HTML.
While native Rust offers more control, Anchor accelerates the development process and reduces the amount of boilerplate code needed to get started. For most developers, Anchor is the recommended framework for building on Solana.
Testing Your Solana Programs
Testing is a crucial part of the development process. Solana offers several testing frameworks depending on your language preference:
- Rust:
solana-program-test
- Typescript:
solana-bankrun
- Python:
bankrun
These frameworks allow you to write and execute tests to ensure your programs work as expected before deploying them to the live network.
Developer Environments on Solana
Mainnet Beta
Mainnet Beta is Solana's production network where all transactions are live and cost real money. It's the environment where your final program will be deployed once it's ready for public use.
Devnet
Devnet is Solana's quality assurance network, similar to a staging environment in traditional development. It's where you deploy your programs for testing in a near-production environment before moving them to Mainnet Beta.
Local Environment
The local environment, set up using solana-test-validator
, is the first environment you should use when developing your programs. It allows you to test your code in isolation before moving to more public environments like Devnet or Mainnet Beta.
Building by Example: Resources and Guides
Solana Cookbook
The Solana Cookbook is a collection of references and code snippets designed to help you build on Solana. It's a valuable resource for both new and experienced developers, offering practical examples and best practices for Solana development.
Solana Program Examples
The Solana Program Examples repository provides a collection of example programs that serve as building blocks for different actions on your programs. These examples can help you get started quickly and understand the basic principles of Solana development.
Solana Guides
Solana Guides are tutorials and walkthroughs that cover various aspects of Solana development. Whether you're building your first onchain program or optimizing your dApp, these guides offer step-by-step instructions to help you succeed.
Frequently Asked Questions
What programming languages can I use for Solana development?
For onchain development, you can use Rust, C, or C++. For client-side development, you can use any language you're comfortable with, such as TypeScript, Python, Java, etc.
Do I need to learn Rust to develop on Solana?
If you're focusing on onchain program development, learning Rust is highly recommended. However, for client-side development, you can use other languages like TypeScript or Python.
What is the Solana CLI, and why do I need it?
The Solana CLI is a command-line tool that allows you to compile, deploy, and manage your onchain programs. It's essential for interacting with the Solana network and testing your programs locally.
Can I test my Solana programs locally?
Yes, you can test your programs locally using the solana-test-validator
, which sets up a local version of the Solana network on your machine.
What is the difference between native Rust and Anchor on Solana?
Native Rust offers more control but requires more boilerplate code. Anchor simplifies the development process with a higher-level API, making it easier and faster to build on Solana.