- Published on
Creating Smart Contracts on the Ethereum Blockchain
- Authors
- Name
- The Alchemist
- @dnwemedia
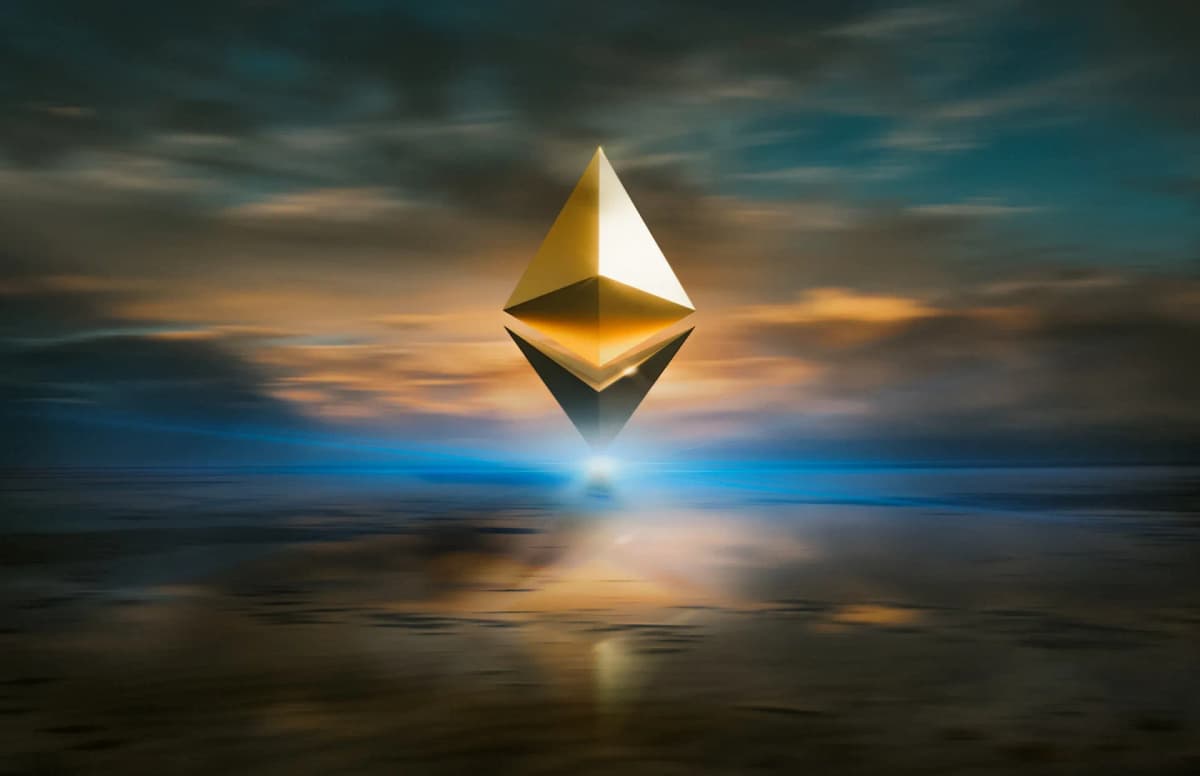
In the ever-evolving landscape of blockchain technology, Ethereum stands out as a pioneer. It's not just a cryptocurrency; it's a platform that enables the creation of decentralized applications (DApps) through smart contracts. Smart contracts have gained immense popularity due to their ability to automate processes and execute agreements without intermediaries. In this comprehensive guide, we will delve into the world of smart contracts on the Ethereum blockchain, exploring what they are, how to create them, and their real-world applications.
Understanding Smart Contracts
What Are Smart Contracts?
Smart contracts are self-executing contracts with the terms of the agreement directly written into code. These contracts run on the Ethereum blockchain, making them immutable and tamper-proof. They automatically execute actions when predefined conditions are met, eliminating the need for intermediaries and enhancing trust in the process.
The Ethereum Blockchain
Ethereum, often referred to as the world computer, is a decentralized platform that allows developers to build DApps and smart contracts. It uses blockchain technology, which is a distributed ledger, to record and verify transactions across a network of computers. Ethereum's blockchain is the foundation on which smart contracts are created and executed.
Creating Your First Smart Contract
Prerequisites
Before you embark on creating a smart contract on the Ethereum blockchain, you'll need the following:
Ethereum Wallet: To interact with the Ethereum network, you'll need a wallet. Popular options include MetaMask and MyEtherWallet.
Solidity: Solidity is the programming language used for writing Ethereum smart contracts. Familiarize yourself with its syntax and structure.
Ethereum Testnet: For testing purposes, it's advisable to use an Ethereum testnet like Ropsten to avoid real Ether transactions.
Writing the Smart Contract
Let's create a simple smart contract that acts as a digital vending machine. It dispenses an item when a certain amount of Ether is sent to it. Below is a basic example written in Solidity:
pragma solidity ^0.8.0;
contract VendingMachine {
address public owner;
uint256 public itemPrice;
uint256 public itemsAvailable;
event ItemPurchased(address indexed buyer);
constructor(uint256 _price, uint256 _quantity) {
owner = msg.sender;
itemPrice = _price;
itemsAvailable = _quantity;
}
function purchaseItem() public payable {
require(msg.value == itemPrice, "Please send the correct amount of Ether.");
require(itemsAvailable > 0, "Item is out of stock.");
// Transfer item to the buyer
// Reduce the item count
// Emit an event
}
}
Deploying the Smart Contract
After writing the smart contract, you need to deploy it to the Ethereum blockchain. This is done through a process called "contract deployment." It involves submitting your contract's bytecode and constructor parameters to the Ethereum network.
Interacting with Your Smart Contract
Once deployed, you can interact with your smart contract through transactions. Users can send Ether to the contract to trigger its functions. In the case of our vending machine, they can purchase items by sending the correct amount of Ether.
Real-World Applications of Smart Contracts
Smart contracts have a wide range of applications across various industries. Some notable examples include:
- Supply Chain Management: Smart contracts can be used to track the movement of goods, ensuring transparency and reducing fraud.
- Finance and Banking: Automated loan agreements, insurance claims processing, and even decentralized finance (DeFi) protocols rely on smart contracts.
- Real Estate: Property transactions can be simplified and made more secure through smart contracts that handle payments and ownership transfers.
- Healthcare: Managing patient records and ensuring privacy can be achieved using blockchain-based smart contracts.
- Legal: Smart contracts can automate legal agreements, such as wills and intellectual property contracts.
Conclusion
In conclusion, smart contracts on the Ethereum blockchain have revolutionized the way we conduct transactions and automate processes. They offer a secure, efficient, and trustless way of executing agreements and managing various aspects of business and everyday life. As you embark on your journey to create smart contracts, remember that continuous learning and practice are key to mastering this transformative technology. So, get coding, experiment, and unlock the full potential of Ethereum smart contracts!